4人まで参加してマルチプレイ出来ます。一人でもタイムアタックで遊べます。AI車は8台で3台が早いです。ほかの人がいる時抜けてもゲームは維持されます。
最初はAI車が箱型でした。2回目のアップデートでPhoton Unity Network(PUN2)にして、free素材の車をAI車に使いました。3回目のアップデートで他の人の入退室を分かりやすくして、マップ表示を付けスマホ対応にしました。今回でアップデートは4回目です。今回4人参加中でも、その内の2人で2周レースができるようにしました。AI車の動きを滑らかにして、他の人の車の上に目印を付け位置の掌握を可能にしました。
ゲーム説明
PC用:キーボードの カーソルキー「→」、「←」で左右にハンドルを切ります。 カーソルキー「↑」、「Z」でアクセル「↓」、「X」でブレーキ、「M」で2人マッチレースです。
スマホ用:「Left」「Right」ボタンで左右にハンドルを切り、「Accel」「Break」ボタンでアクセル、ブレーキ、「Match」ボタンで2人マッチレースです。
AI車にはStandard AssetsにあったWaypointCircuitを使っていますが、現在Standard Assetsはダウンロードできなくなってしまいました。しかし、GitHubからWaypointCircuit.csとWaypointProgressTracker.csの2つをダウンロードしてWaypointProgressTracker.csのvoid Start()にcircuit = GameObject.FindWithTag(“waypoint”).GetComponent();を追加すれば使えます。
private void Start()
{
circuit = GameObject.FindWithTag("waypoint").GetComponent<WaypointCircuit>();
if (target == null)
{
target = new GameObject(name + " Waypoint Target").transform;
}
Reset();
}
https://github.com/unity3d-jp/playgrownd/blob/master/Assets/Standard%20Assets/Utility/WaypointCircuit.cs
https://github.com/adametry/HackHeist/blob/master/Assets/Standard%20Assets/Utility/WaypointProgressTracker.cs
使い方は、WaypointCircuitで検索して下さい。だだ3Dでルートを指定するため目標地点のWaypointsと移動させるオブジェクトの高さを合わせなければ、地面にめり込んでしまうかもしれません。かなり癖のあるアセットですが、便利です。
最初はAI車が箱型
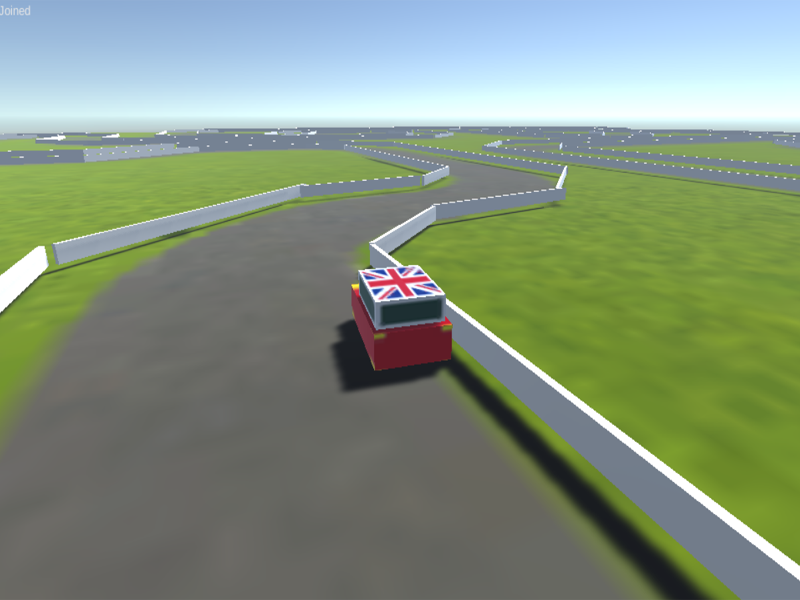
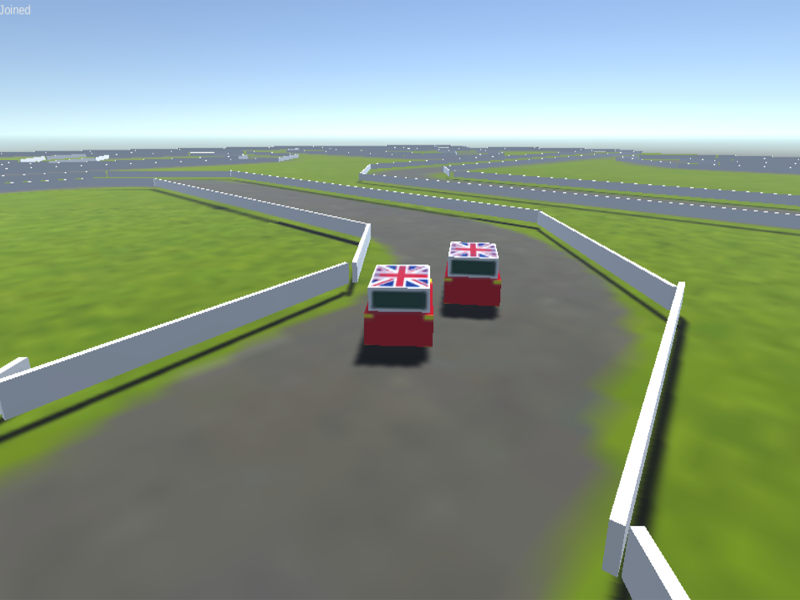
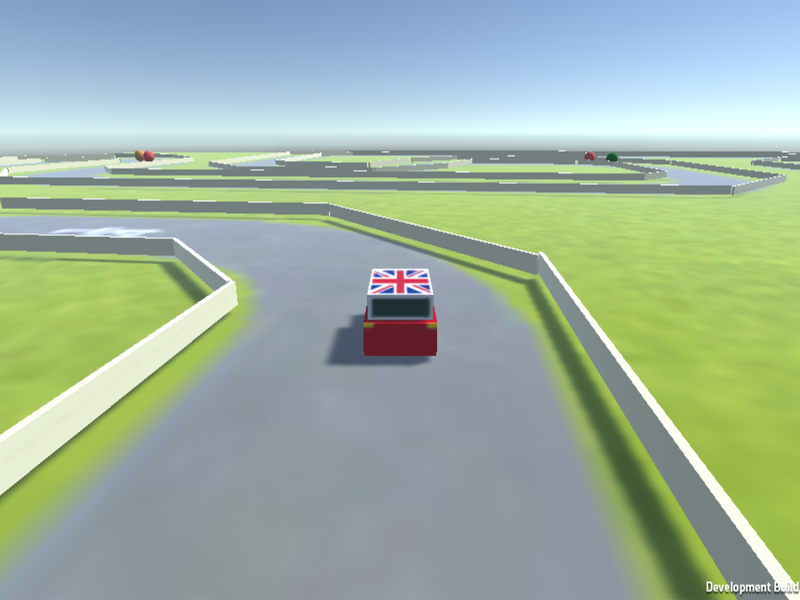
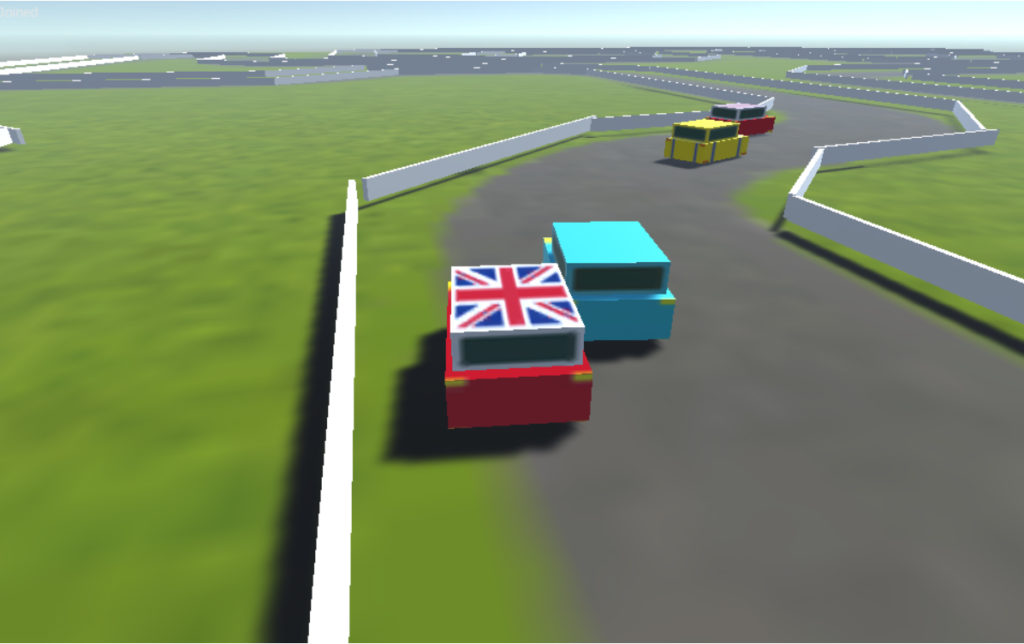
2回目のアップデート(free素材のAI車)
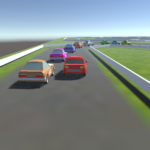
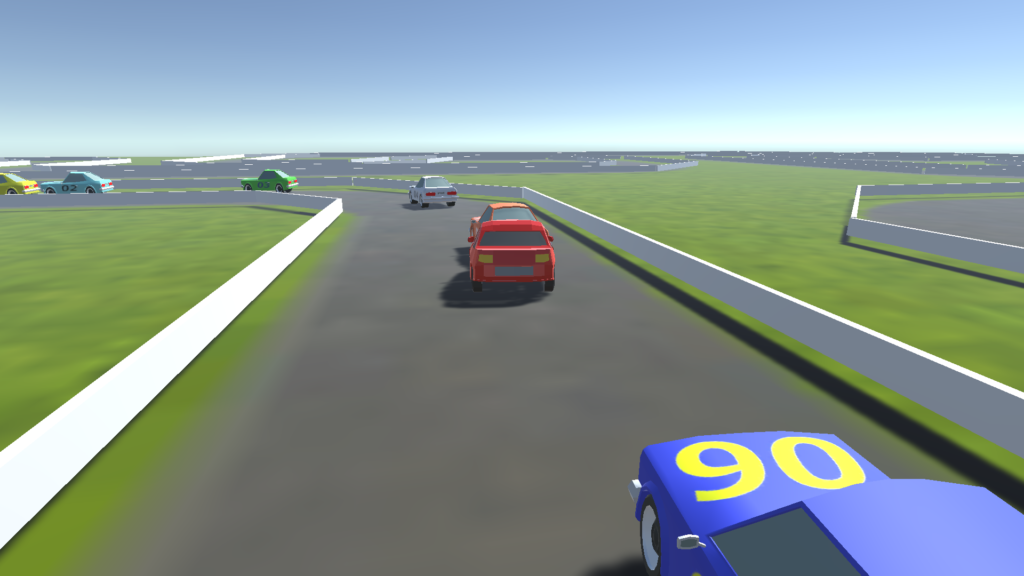
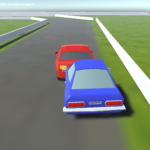
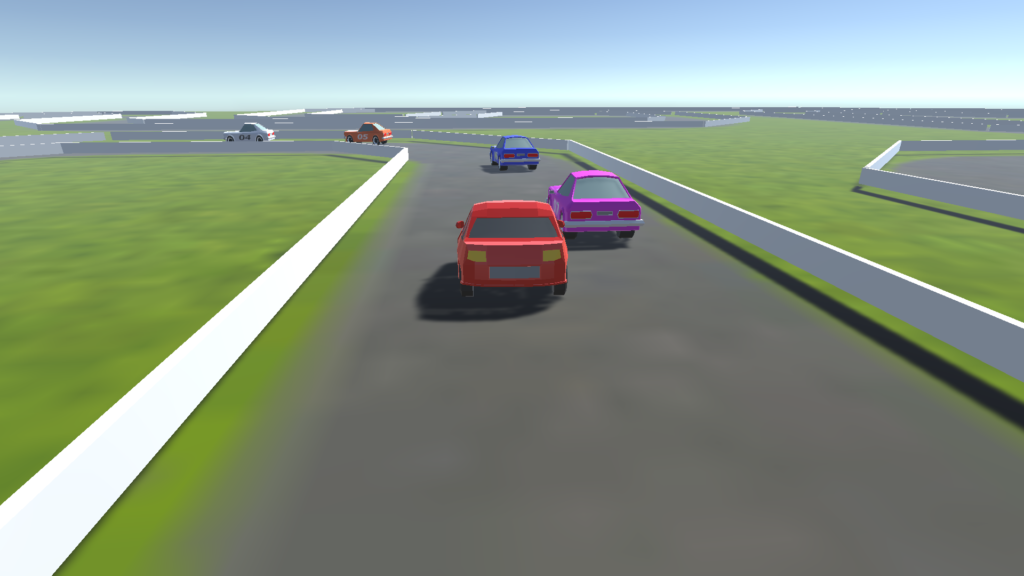
3回目のアップデート(他の人の入退室を分かりやすくマップも表示)
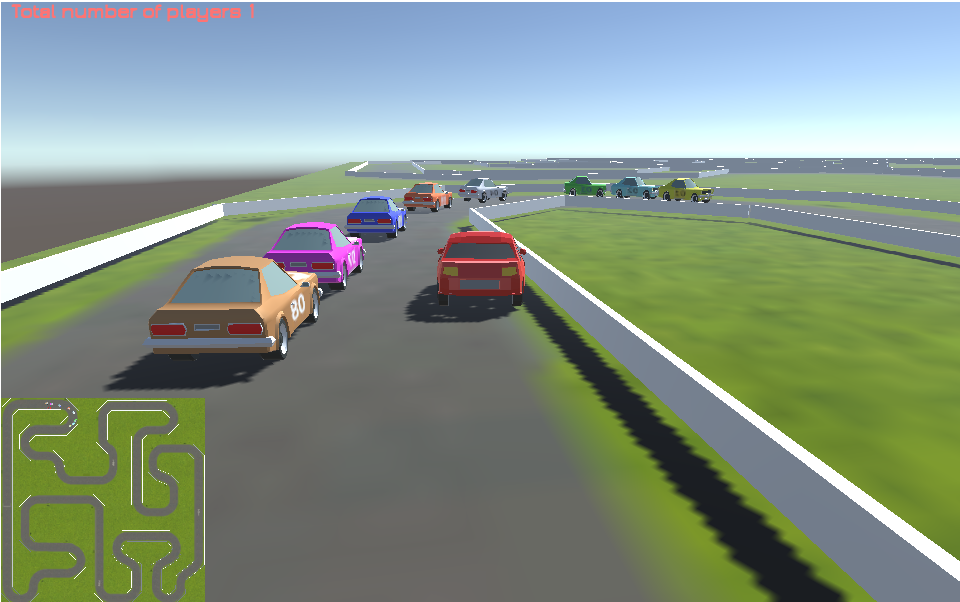
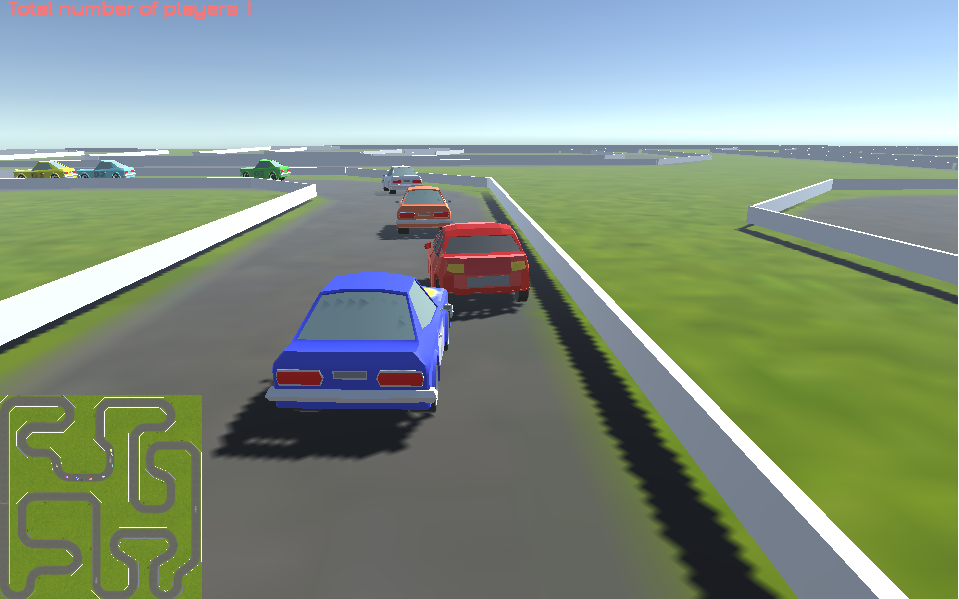
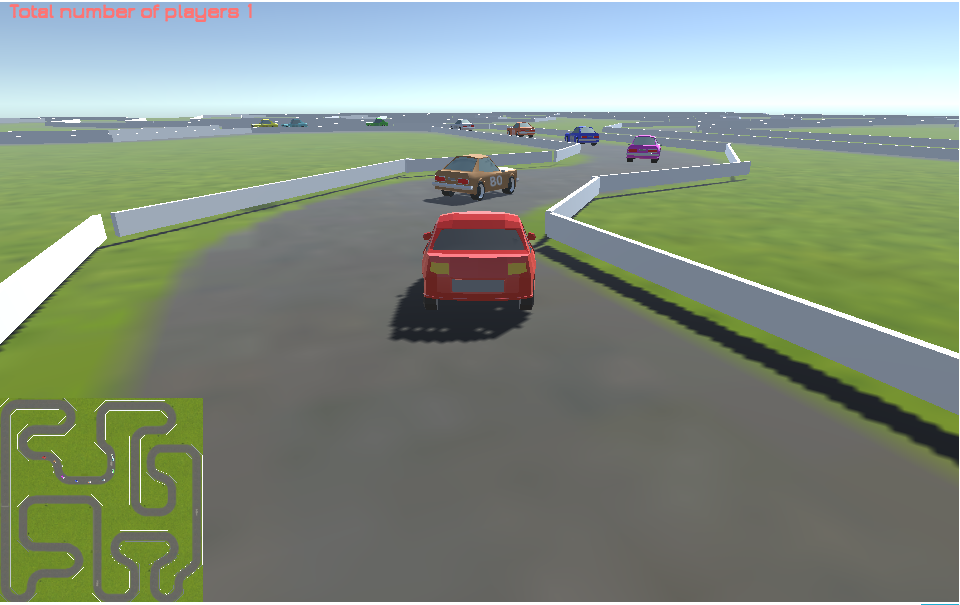
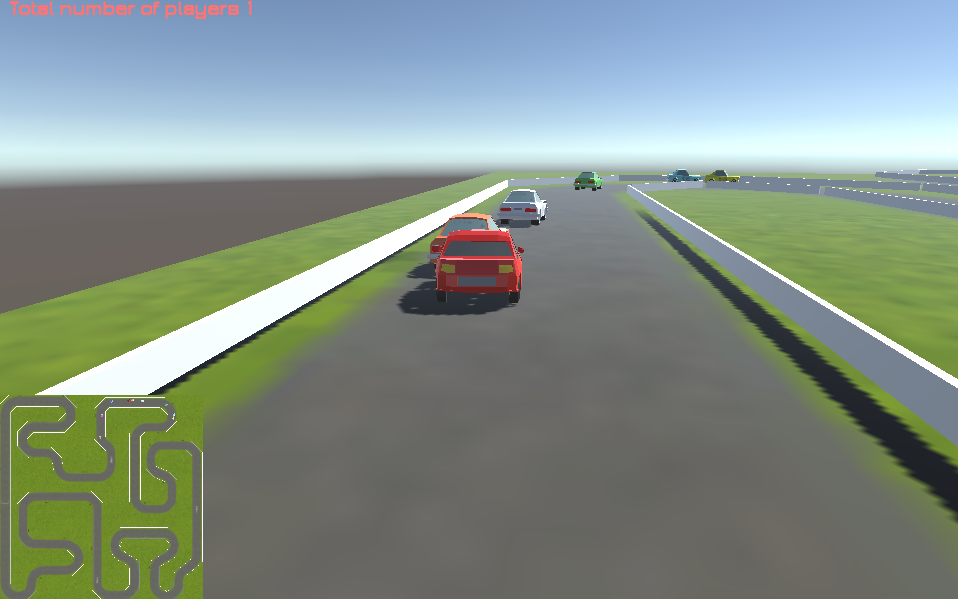
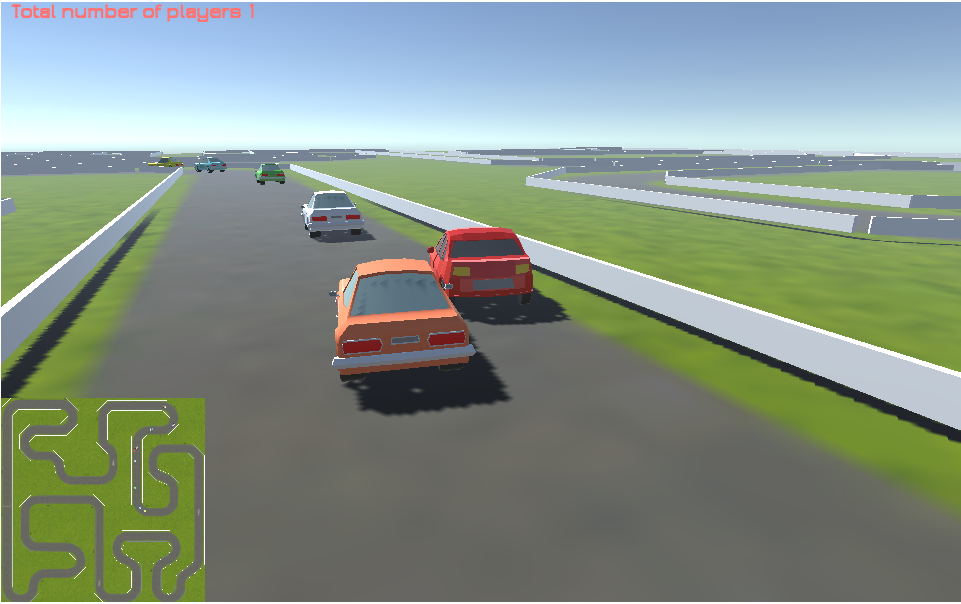
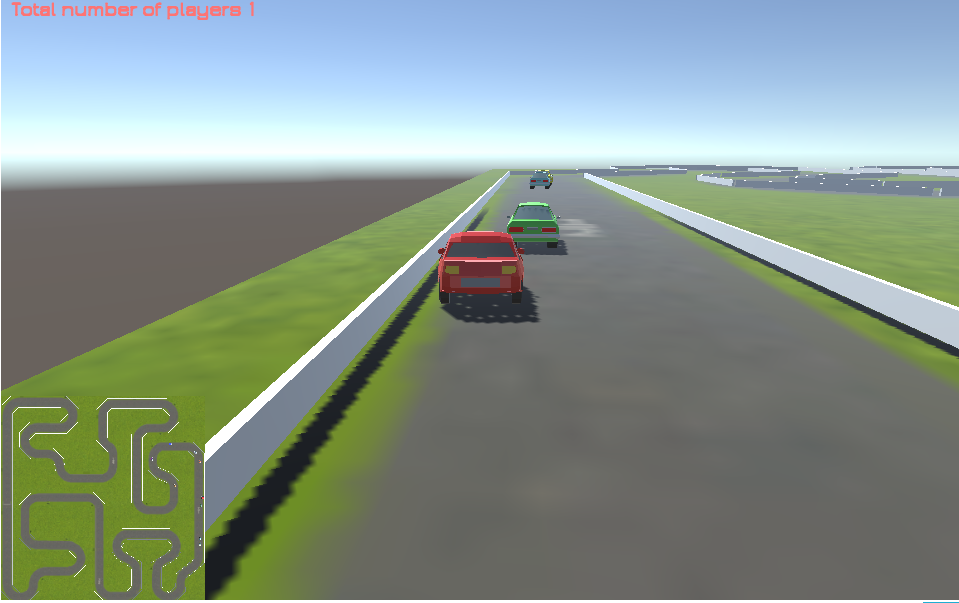
4回目のアップデート(2人の車で2周レースができる)
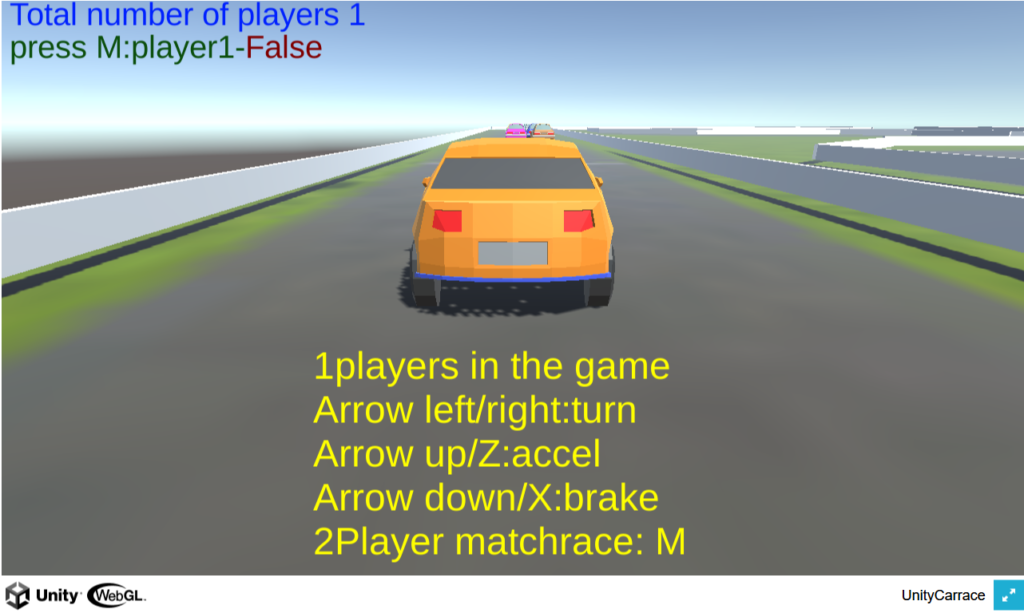
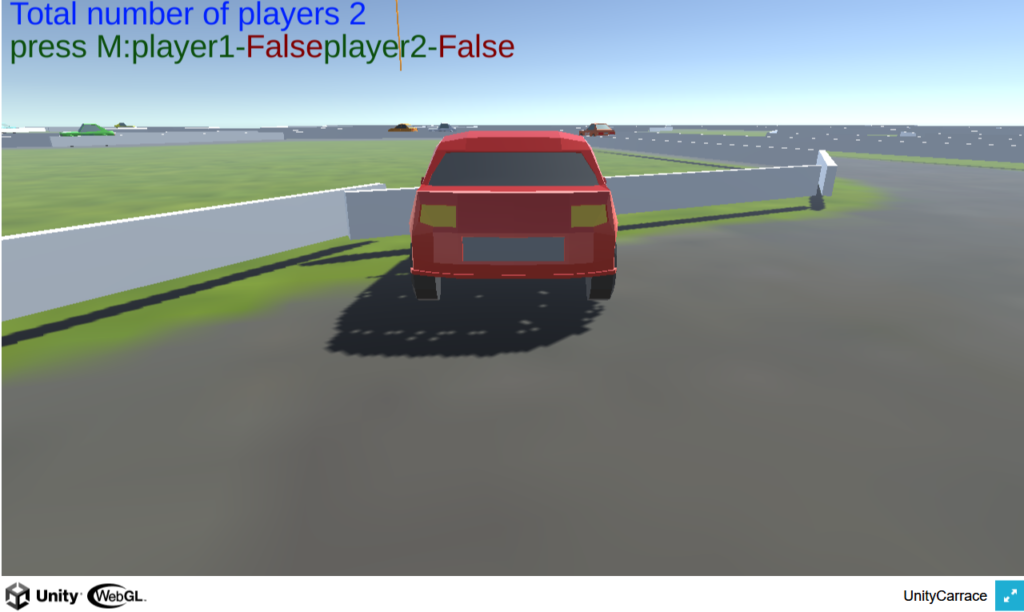
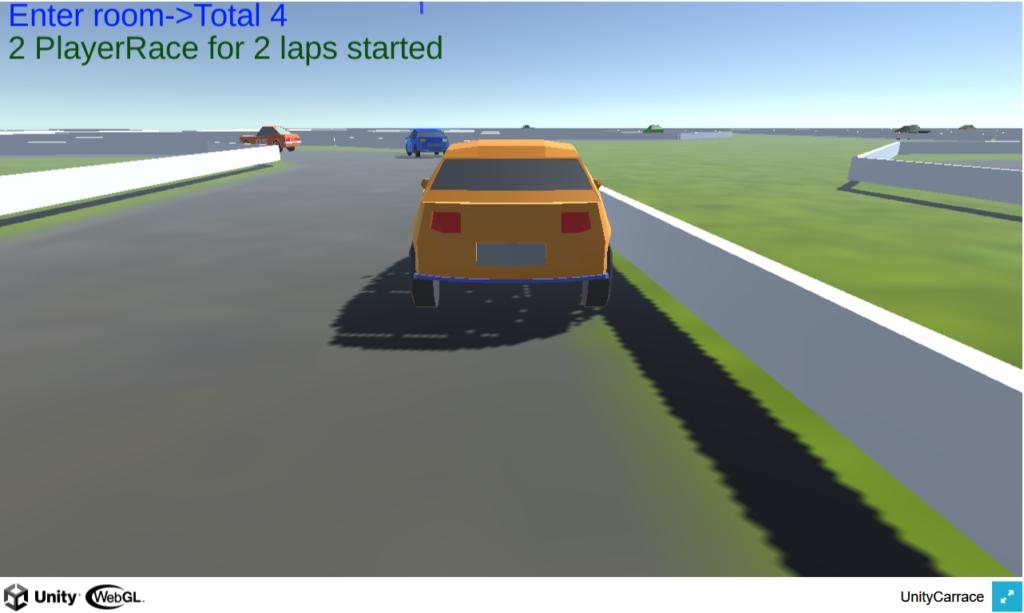
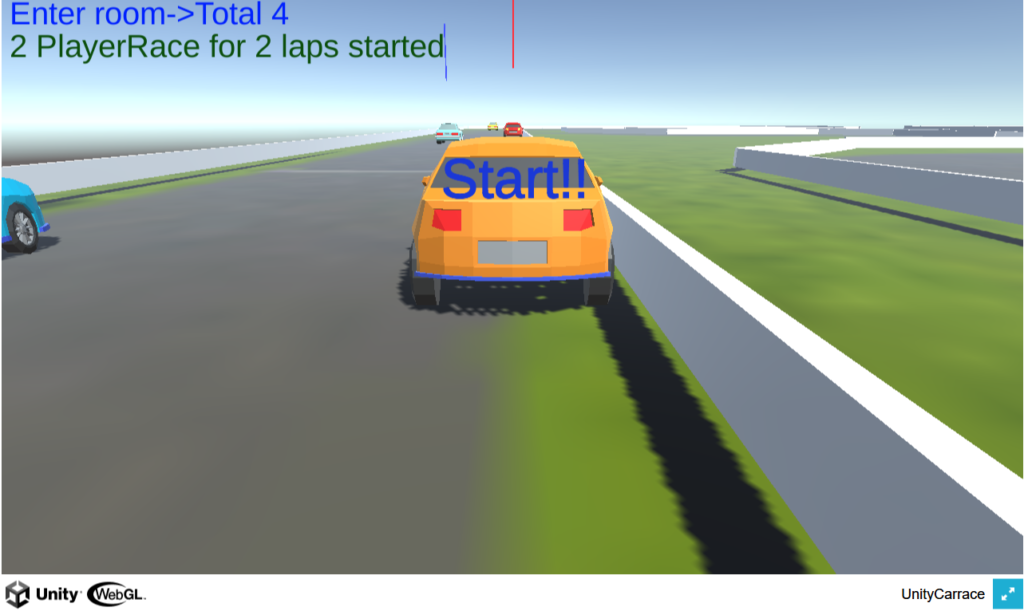
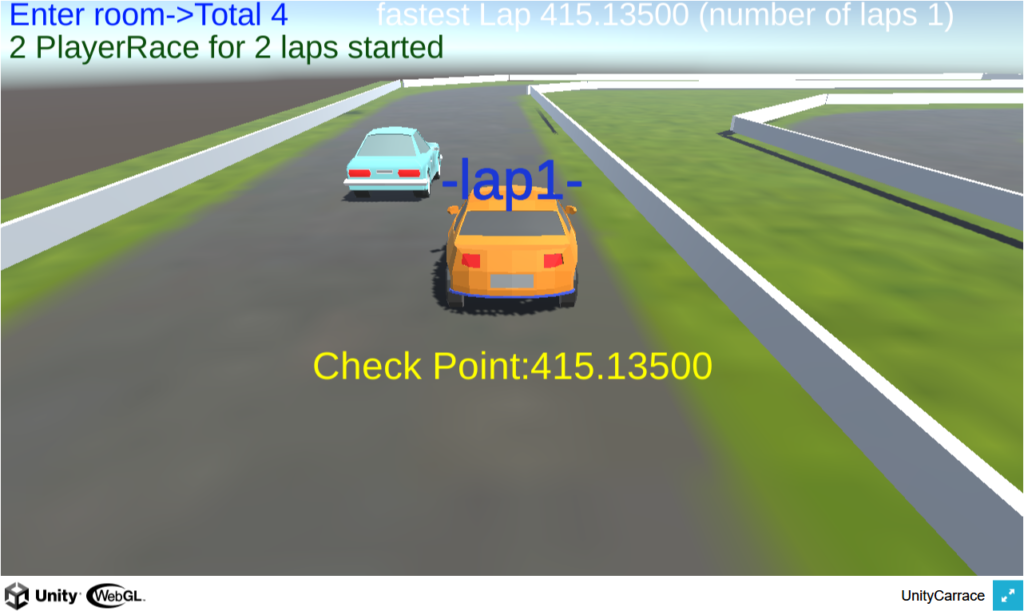
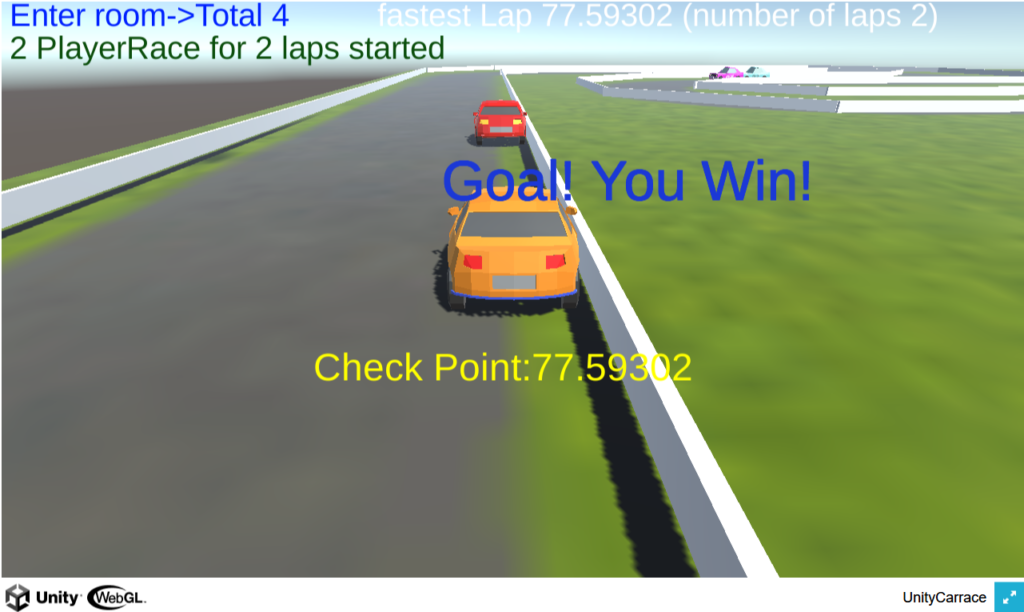
コメント